Chakra UI and React
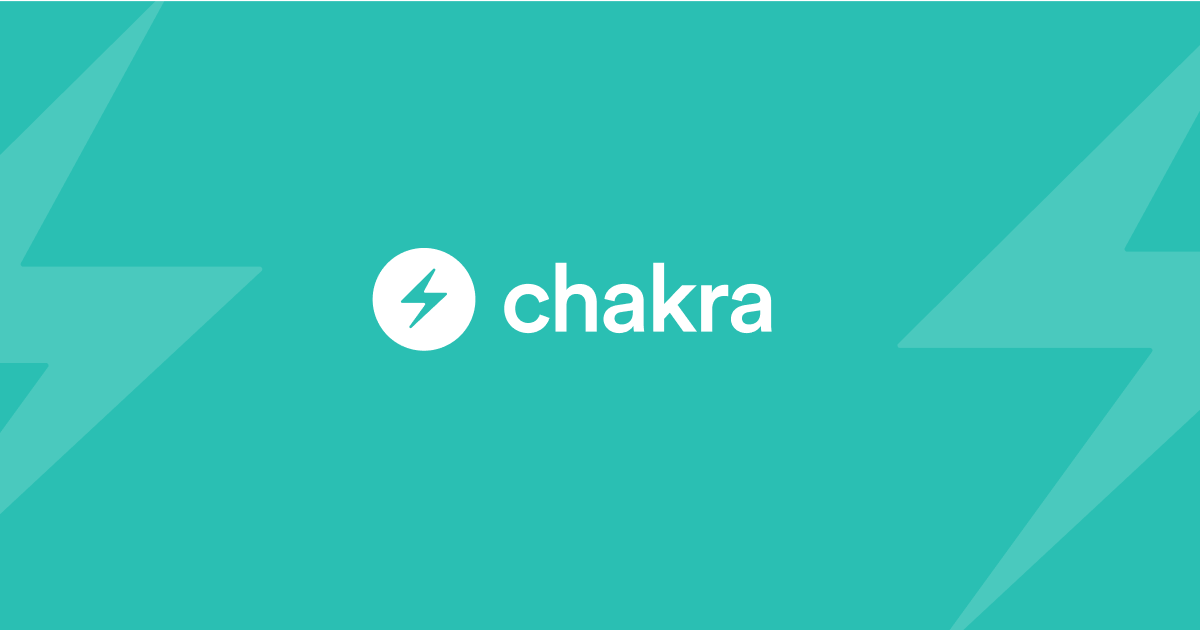
Chakra UI is a powerful tool in the hands of a developer when working with React. In this article we take a look at it.
Introduction
React is a JavaScript library for building user interfaces, and Chakra UI is a library of reusable React components that uses the power of styled-components and the emotion-theming library to easily style your application. Together, these two libraries allow you to build fast, scalable, and accessible websites.
In this tutorial, we will go over the steps to build a basic website using Chakra UI and React. We will start by setting up a new React project and installing the necessary dependencies, then we will create a simple layout using Chakra UI components. Finally, we will add some content to the website and deploy it to the web.
One of the main advantages of Chakra UI is its focus on accessibility. The library includes built-in support for keyboard navigation, screen reader support, and semantic HTML. This makes it easy to build applications that are accessible to users with disabilities, which is important for creating an inclusive and user-friendly experience.
Another benefit of Chakra UI is its simplicity. The library provides a set of concise, well-documented components that are easy to use and customize. This can make it a good choice for developers who want to get up and running quickly without spending much time learning a complex API.
In addition to its accessibility and simplicity, Chakra UI is also highly customizable. It includes a flexible theming system that allows you to quickly change the look and feel of your application. This can be particularly useful for building applications that need to match a specific brand or design language.
Prerequisites
Before getting started, you will need to have the following tools installed on your machine:
Step 1: Setting up the project
The first step is to create a new React project and install the necessary dependencies. You can do this using the create-react-app
tool.
Open a terminal and run the following command:
npx create-react-app my-website
This will create a new directory called my-website with a basic React setup.
Next, navigate to the project directory and install Chakra UI and the @emotion/core library:
cd my-website
npm install @chakra-ui/react @emotion/core
Step 2: Creating the layout
Now that we have a basic React project set up, we can start building the layout of our website using Chakra UI components.
First, open src/App.js
and import the Chakra UI components and the css
function from the @emotion/core
library:
import { Box, Flex, Text, Link } from '@chakra-ui/react';
import { css } from '@emotion/core';
Then, replace the default JSX code with the following:
function App() {
return (
<Box
css={css`
min-height: 100vh;
`}
>
<Flex
as="header"
align="center"
justify="space-between"
p={5}
bg="teal.500"
color="white"
>
<Text fontSize="xl">My Website</Text>
<Box>
<Link href="#" color="white">
Home
</Link>
<Link href="#" color="white" ml={2}>
About
</Link>
<Link href="#" color="white" ml={2}>
Contact
</Link>
</Box>
</Flex>
<Box p={5}>
<Text fontSize="xl">Welcome to my website!</Text>
</Box>
</Box>
);
}
This will create a simple header with a navigation menu and a welcome message in the main content area.
Step 3: Adding content
Now that we have a basic layout for our website, we can start adding some content.
First, let's create a new component for the home page. In the src
directory, create a new file called Home.js
and add the following code:
import React from 'react';
import { Box, Heading, Text } from '@chakra-ui/react';
const Home = () => (
<Box p={5}>
<Heading>Welcome to my website!</Heading>
<Text>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur
pellentesque, libero id scelerisque tincidunt, urna turpis rutrum ante,
non congue ligula justo eu tellus. Nulla facilisi.
</Text>
</Box>
);
export default Home;
This will create a simple component with a heading and some placeholder text.
Next, let's make a new component for the about page. In the src
directory, create a new file called About.js
and add the following code:
import React from 'react';
import { Box, Heading, Text } from '@chakra-ui/react';
const About = () => (
<Box p={5}>
<Heading>About me</Heading>
<Text>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur
pellentesque, libero id scelerisque tincidunt, urna turpis rutrum ante,
non congue ligula justo eu tellus. Nulla facilisi.
</Text>
</Box>
);
export default About;
This will create another simple component with a heading and some placeholder text.
Finally, let's create a new component for the contact page. In the src
directory, create a new file called Contact.js
and add the following code:
import React from 'react';
import { Box, Heading, Text } from '@chakra-ui/react';
const Contact = () => (
<Box p={5}>
<Heading>Contact me</Heading>
<Text>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur
pellentesque, libero id scelerisque tincidunt, urna turpis rutrum ante,
non congue ligula justo eu tellus. Nulla facilisi.
</Text>
</Box>
);
export default Contact;
This will create another simple component with a heading and some placeholder text.
Now that we have our three-page components let's update the App
component to render the correct page based on the current route.
First, import the Home
, About
, and Contact
components at the top of App.js
:
import Home from './Home';
import About from './About';
import Contact from './Contact';
Next, we need to update the App
component to use the react-router-dom
library to handle routing.
First, install the library by running the following command in your terminal:
npm install react-router-dom
Then, import the BrowserRouter
, Route
, and Link
components from react-router-dom
in App.js
:
import { BrowserRouter, Route, Link } from 'react-router-dom';
Now we can update the JSX code in the App
component to use the BrowserRouter
component and the Route
component to render the correct page based on the current route:
function App() {
return (
<BrowserRouter>
<Box
css={css`
min-height: 100vh;
`}
>
<Flex
as="header"
align="center"
justify="space-between"
p={5}
bg="teal.500"
color="white"
>
<Text fontSize="xl">My Website</Text>
<Box>
<Link to="/" color="white">
Home
</Link>
<Link to="/about" color="white" ml={2}>
About
</Link>
<Link to="/contact" color="white" ml={2}>
Contact
</Link>
</Box>
</Flex>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Box>
</BrowserRouter>
);
}
This will update the App
component to use the react-router-dom
library to handle routing and render the correct page based on the current route.
Step 4: Deploying the website
Now that we have a basic website with Chakra UI and React, we can deploy it to the web. There are many ways to do this, but one easy way is to use the Vercel hosting platform.
First, create a Vercel account and install the Vercel CLI:
npm install -g vercel
Then, navigate to the root directory of your project and run the following command to deploy the website:
vercel
This will build and deploy the website to Vercel, and provide you with a URL to access it.
Conclusion
This tutorial taught us how to build a basic website with Chakra UI and React. We set up a new React project and installed the necessary dependencies, created a simple layout using Chakra UI components, added some content to the website, and deployed it to the web. With these skills, you should now be able to build your websites using Chakra UI and React and expand further from this article.